Description
While developing a typescript (TS) application, unit testing is needed. Also, unit tests are written in TS.
Jasmine is the test framework used to test TS code.
Jasmine standalone is used to execute unit tests, which are executed in node, without browser.
All files with unit tests are in spec
folder, with *.spec.ts
extension.
The structure of spec
folder should be similar to the structure of src
folder (where is code).
Jasmin standalone configuration is in spec\support\jasmine.json
.
Unit tests are executed with npm test
, or from vscode Test Explorer UI extension.
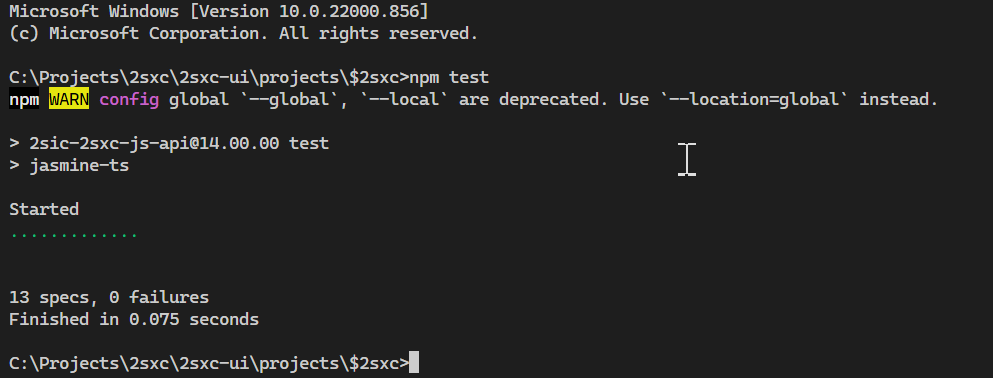
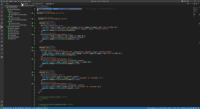
Pre req
- .gitignore, git init; git add .; git commit
npm init
npm install --save-dev typescript
npx tsc --init
- tsconfig.json
{"compilerOptions":
{"target": "es2016",
"module": "commonjs",
"lib": ["es2016","dom"],
"allowJs": true,
"sourceMap": true,
"rootDir": "./src",
"strict": true,
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true
}
}
npm install --save-dev webpack webpack-cli webpack-dev-server
npm install --save-dev ts-loader
- webpack.config.js
const path = require('path');
module.exports = {
entry: './src/index.ts',
devtool: 'inline-source-map',
module: {
rules: [
{
test: /\.tsx?$/,
use: 'ts-loader',
exclude: /node_modules/,
include: [
path.resolve(__dirname, "src")
],
},
],
},
resolve: {
extensions: [ '.tsx', '.ts', '.js' ],
},
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist'),
},
};
- add script to package.json
"build": "npx webpack --config=webpack.config.js"
npm run build
Jasmine standalone
npm install --save-dev jasmine @types/jasmine
npm install --save-dev ts-node jasmine-ts
npm install --save-dev jasmine-spec-reporter
- jasmine.json
{
"reporters": [
{
"name": "jasmine-spec-reporter#SpecReporter",
"options": {
"displayStacktrace": "all"
}
}
],
"spec_dir": "spec",
"spec_files": ["**/*.[sS]pec.ts"]
}
- add script to package.json
"test": "jasmine-ts"
npm test
More info
https://jasmine.github.io/pages/getting_started.html